Using TradingView Strategy Alert Messages for Tick and Percentage Based Stops & Targets
Explore how to automate trading by sending TradingView alerts with fixed or dynamic stop-loss and take-profit levels to NinjaTrader using CrossTrade.
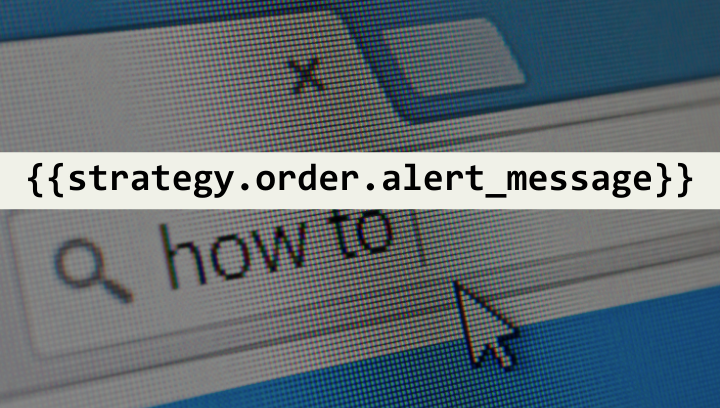
Last month, we released new functionality to set Take Profit and Stop Loss price level limit orders on NinjaTrader in a single alert.
Most NinjaTraders are familiar with using ATM (Advanced Trade Management) strategies for bracket orders, which are easy to send in an alert. ATMs can set TP/SL based on ticks or percentages.
But what if you want to set profit and stop loss levels based on your TV strategy when NinjaTrader only accepts price values for limit orders outside of an ATM?
In this article, we'll explore how to send alerts from TradingView to NinjaTrader via CrossTrade, focusing on strategies with fixed stops and targets based on both tick and percentage values.
Why Use CrossTrade?
CrossTrade acts as a bridge between TradingView and NinjaTrader, enabling seamless automated bot trading. With CrossTrade, you can take advantage of TradingView’s powerful charting and alerting features and execute trades directly in NinjaTrader. This is particularly useful for traders who want to automate their trading strategies without relying solely on NinjaTrader's internal scripting capabilities.
Step 1: Creating a Strategy in TradingView
To begin, you need to create a strategy in TradingView. We will explore two versions: one that uses fixed stops and targets based on tick values, and another that uses percentage-based stops and targets.
Tick-Based Stops and Targets
In futures trading, ticks represent the smallest price movement an asset can make. For example, in the NQ (Nasdaq 100 E-mini) and ES (S&P 500 E-mini) futures, a tick is equal to 0.25 points. The following Pine Script strategy uses fixed stops and targets based on a 50-tick move:
Download directly on TradingView
//@version=5
strategy("SMA Crossover with 50 Tick SL/TP", overlay=true)
// Define the SMAs
sma1 = ta.sma(close, 1)
sma3 = ta.sma(close, 3)
// Define the crossover conditions
longCondition = ta.crossover(sma1, sma3)
shortCondition = ta.crossunder(sma1, sma3)
// Define tick sizes for NQ and ES
tickSizeNQ = 0.25
tickSizeES = 0.25
// Initialize the tickSize variable with a float type
var float tickSize = na
// Determine the current symbol's tick size
if (syminfo.ticker == "NQ" or syminfo.root == "NQ")
tickSize := tickSizeNQ
else if (syminfo.ticker == "ES" or syminfo.root == "ES")
tickSize := tickSizeES
// Calculate stop loss and target prices based on 50 ticks
if not na(tickSize)
stopLossTicks = 50 * tickSize
targetTicks = 50 * tickSize
if longCondition
stopLossPrice = close - stopLossTicks
targetPrice = close + targetTicks
alertMessage = 'key=your-secret-key;'
alertMessage += 'command=PLACE;'
alertMessage += 'account=sim101;'
alertMessage += 'instrument=NQ 09-24;'
alertMessage += 'action=BUY;'
alertMessage += 'qty=1;'
alertMessage += 'order_type=MARKET;'
alertMessage += 'tif=DAY;'
alertMessage += 'flatten_first=true;'
alertMessage += 'take_profit=' + str.tostring(targetPrice) + ';'
alertMessage += 'stop_loss=' + str.tostring(stopLossPrice) + ';'
strategy.entry("Long", strategy.long, alert_message=alertMessage)
if shortCondition
stopLossPrice = close + stopLossTicks
targetPrice = close - targetTicks
alertMessage = 'key=your-secret-key;'
alertMessage += 'command=PLACE;'
alertMessage += 'account=sim101;'
alertMessage += 'instrument=NQ 09-24;'
alertMessage += 'action=SELL;'
alertMessage += 'qty=1;'
alertMessage += 'order_type=MARKET;'
alertMessage += 'tif=DAY;'
alertMessage += 'flatten_first=true;'
alertMessage += 'take_profit=' + str.tostring(targetPrice) + ';'
alertMessage += 'stop_loss=' + str.tostring(stopLossPrice) + ';'
strategy.entry("Short", strategy.short, alert_message=alertMessage)
// Plot the SMAs
plot(sma1, color=color.blue, title="1 SMA")
plot(sma3, color=color.red, title="3 SMA")
Percentage-Based Stops and Targets
In some strategies, you might want your stop loss and take profit levels to adjust dynamically based on the market price. A percentage-based approach allows for this flexibility. Here's a version of the same strategy using 1% stops and targets:
Download directly on TradingView
//@version=5
strategy("SMA Crossover with 1% SL/TP", overlay=true)
// Define the SMAs
sma1 = ta.sma(close, 1)
sma3 = ta.sma(close, 3)
// Define the crossover conditions
longCondition = ta.crossover(sma1, sma3)
shortCondition = ta.crossunder(sma1, sma3)
// Define the percentage for stop loss and target
percentage = 1.0
// Calculate stop loss and target prices based on 1% above and below the entry price
if longCondition
stopLossPrice = close * (1 - percentage / 100)
targetPrice = close * (1 + percentage / 100)
alertMessage = 'key=your-secret-key;'
alertMessage += 'command=PLACE;'
alertMessage += 'account=sim101;'
alertMessage += 'instrument=NQ 09-24;'
alertMessage += 'action=BUY;'
alertMessage += 'qty=1;'
alertMessage += 'order_type=MARKET;'
alertMessage += 'tif=DAY;'
alertMessage += 'flatten_first=true;'
alertMessage += 'take_profit=' + str.tostring(targetPrice) + ';'
alertMessage += 'stop_loss=' + str.tostring(stopLossPrice) + ';'
strategy.entry("Long", strategy.long, alert_message=alertMessage)
if shortCondition
stopLossPrice = close * (1 + percentage / 100)
targetPrice = close * (1 - percentage / 100)
alertMessage = 'key=your-secret-key;'
alertMessage += 'command=PLACE;'
alertMessage += 'account=sim101;'
alertMessage += 'instrument=NQ 09-24;'
alertMessage += 'action=SELL;'
alertMessage += 'qty=1;'
alertMessage += 'order_type=MARKET;'
alertMessage += 'tif=DAY;'
alertMessage += 'flatten_first=true;'
alertMessage += 'take_profit=' + str.tostring(targetPrice) + ';'
alertMessage += 'stop_loss=' + str.tostring(stopLossPrice) + ';'
strategy.entry("Short", strategy.short, alert_message=alertMessage)
// Plot the SMAs
plot(sma1, color=color.blue, title="1 SMA")
plot(sma3, color=color.red, title="3 SMA")
Step 2: Setting Up Alerts in TradingView
After creating your strategy, the next step is to set up alerts in TradingView. This process is crucial for triggering trades in NinjaTrader via CrossTrade.
Create an Alert:
- Click on the "Alerts" tab in TradingView and select "Create Alert."
- Choose your strategy as the condition, and set the alert to trigger on "Any alert() function call."
Customize the Alert Message:
In the "Message" field of the alert dialog, input the following: {{strategy.order.alert_message}}
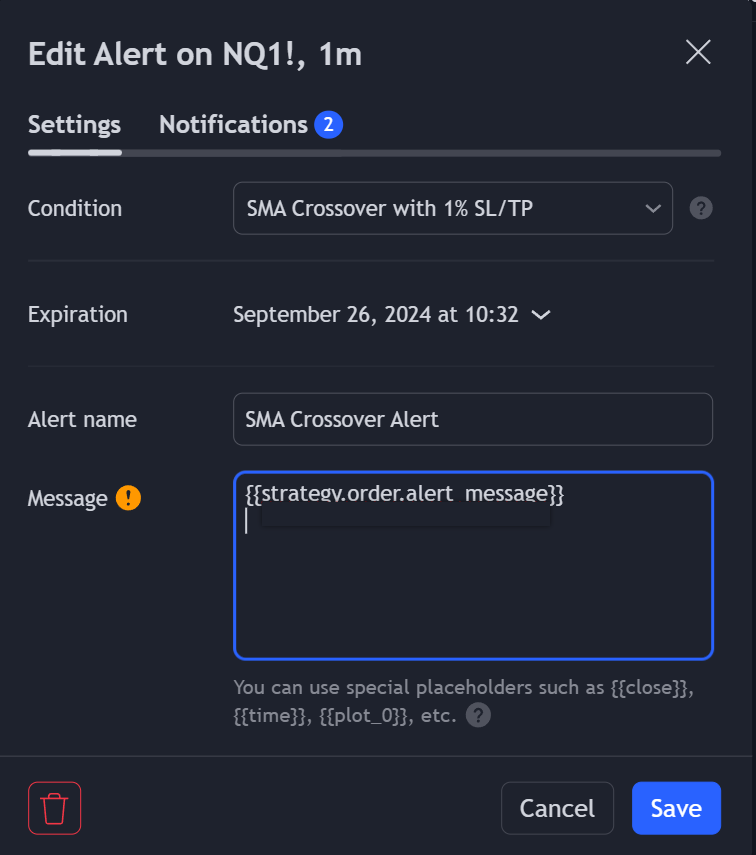
This placeholder ensures that the custom alert message generated by your Pine Script is passed directly to CrossTrade.
Step 3: Sending Alerts to NinjaTrader via CrossTrade
Once your alert is triggered, the message will be sent to CrossTrade via a webhook. CrossTrade then interprets this message and places the corresponding order in NinjaTrader. This process allows you to automate your trading strategy fully, from signal generation in TradingView to order execution in NinjaTrader.
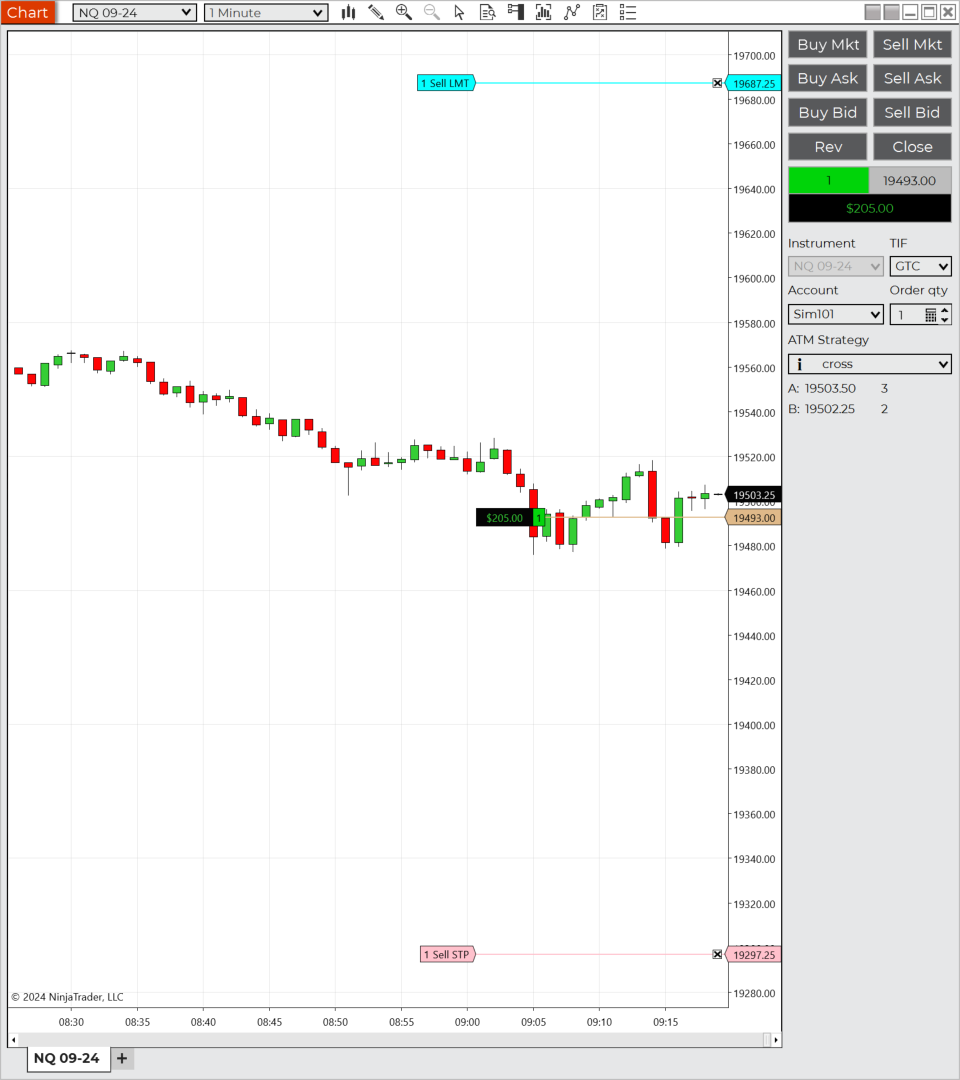
Final thoughts
By combining TradingView, CrossTrade, and NinjaTrader, you can create a powerful automated trading system. Whether you're using fixed stops and targets based on ticks or more dynamic percentage-based levels, the process remains straightforward. The key is to craft the alert message correctly in your Pine Script and use the {{strategy.order.alert_message}}
placeholder in TradingView. With these tools and techniques, you can take full advantage of automated trading and focus more on refining your strategies and less on manual execution.
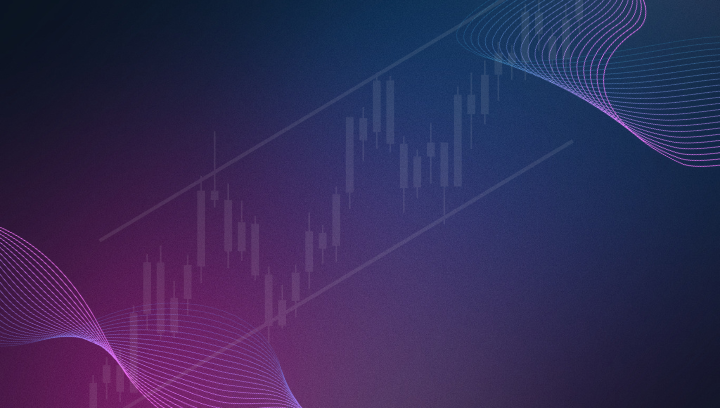